Automating Sequences
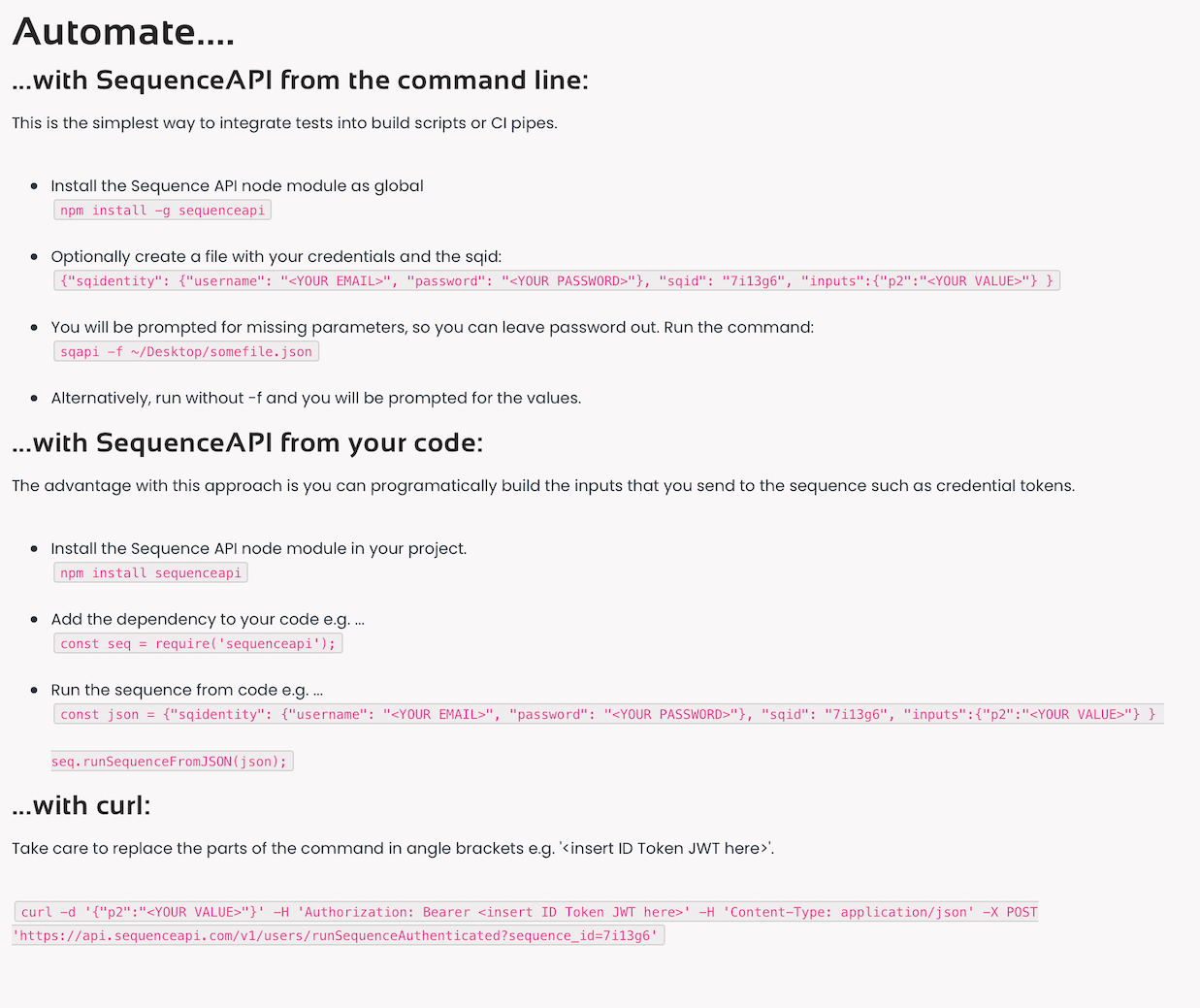
Developing tests, or ad hoc checks on your code as you write it, can be done in a browser. But beyond that, tests need to be automated for continuous integration and deployment (CI/CD). Further, some tests need parameters that are not practical to generate manually each run, and are therefore better run within a script or program. For example, fresh authorisation tokens can be painful to generate outside of code.
Therefore, SequenceAPI provides a node module that offers 3 routes for automation.
- A command line utility (when the node module is installed globally).
- A javascript library that provides a range of utility methods for creating and running sequences.
- Calling the SequenceAPI api directly (aka Curl).
I'm going to use a couple of different sequences to demonstrate automation approaches.
The first is here and simply takes a number you pass in at runtime and adds it to another.
SequenceAPI started life as a side project to help develop/test a data privacy product, built on AWS Lambda, called Jevul. I'm going to use one of the tests we built for Jevul to walk through the automation process as a more cophisticated example.
It's a fairly complex test that checks a series of conditions under which one user can see the data of another user. You can find it here, but this is what it looks like:
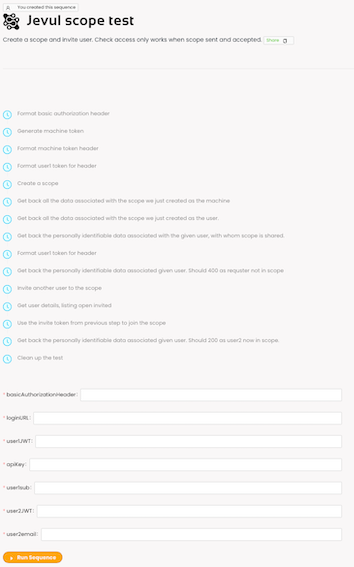
This is a very important test. I run it manually (from the command line utility) when I change relevant code. It runs each night, scheduled as a cron, and against each new staged release. It can't really be run from the browser, because it uses a JSON Web Token that is painful to generate - and besides that's a lot of fields to fill out every time.
I am going to describe in detail how to automate this. But it's worth noting that customized automation pages come with every sequence. Below, I illustrate with the simpler Sequence. If you use the link in the edit page...
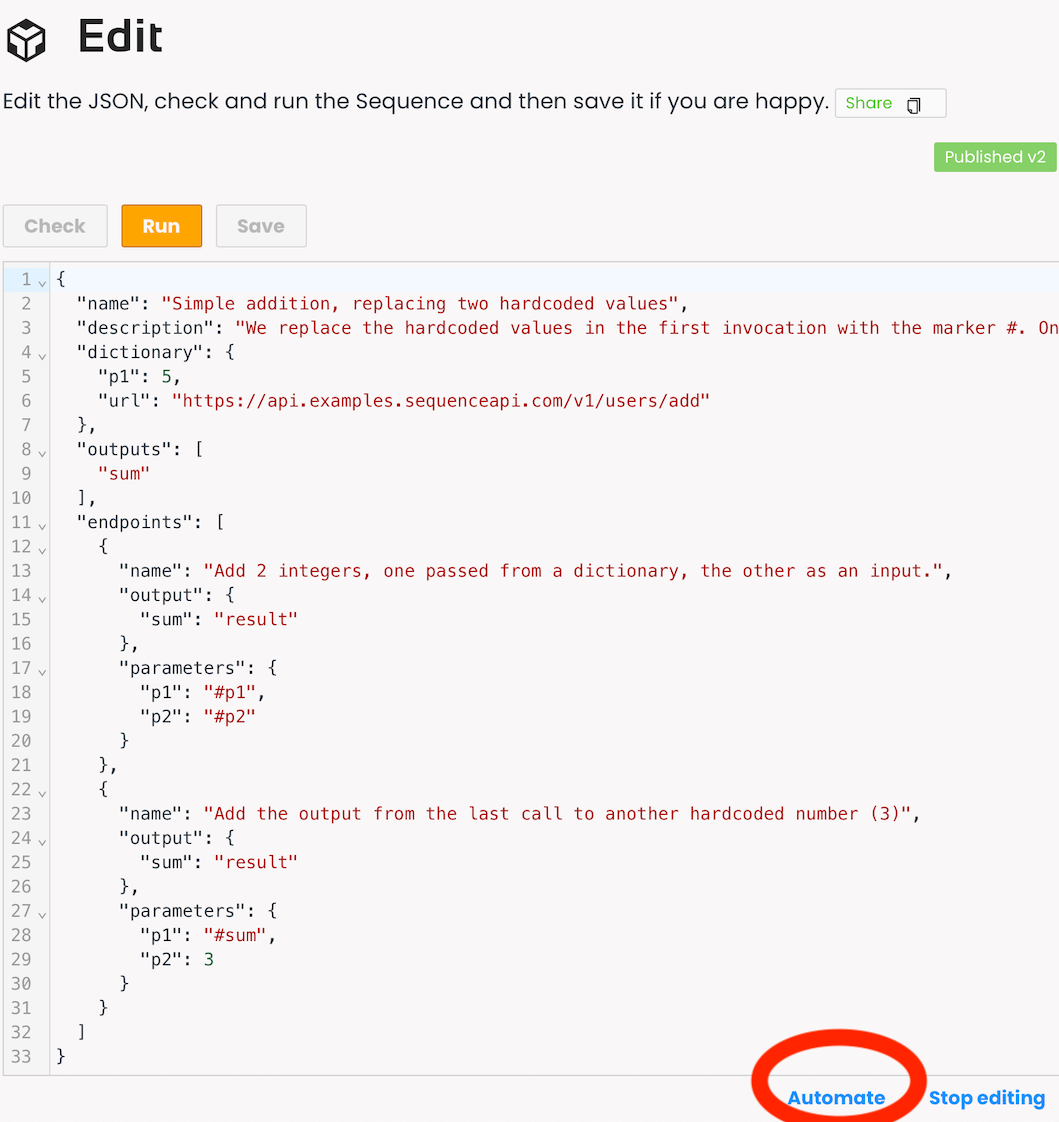
The automation instructions for the sequence we are automating are here and look like this:
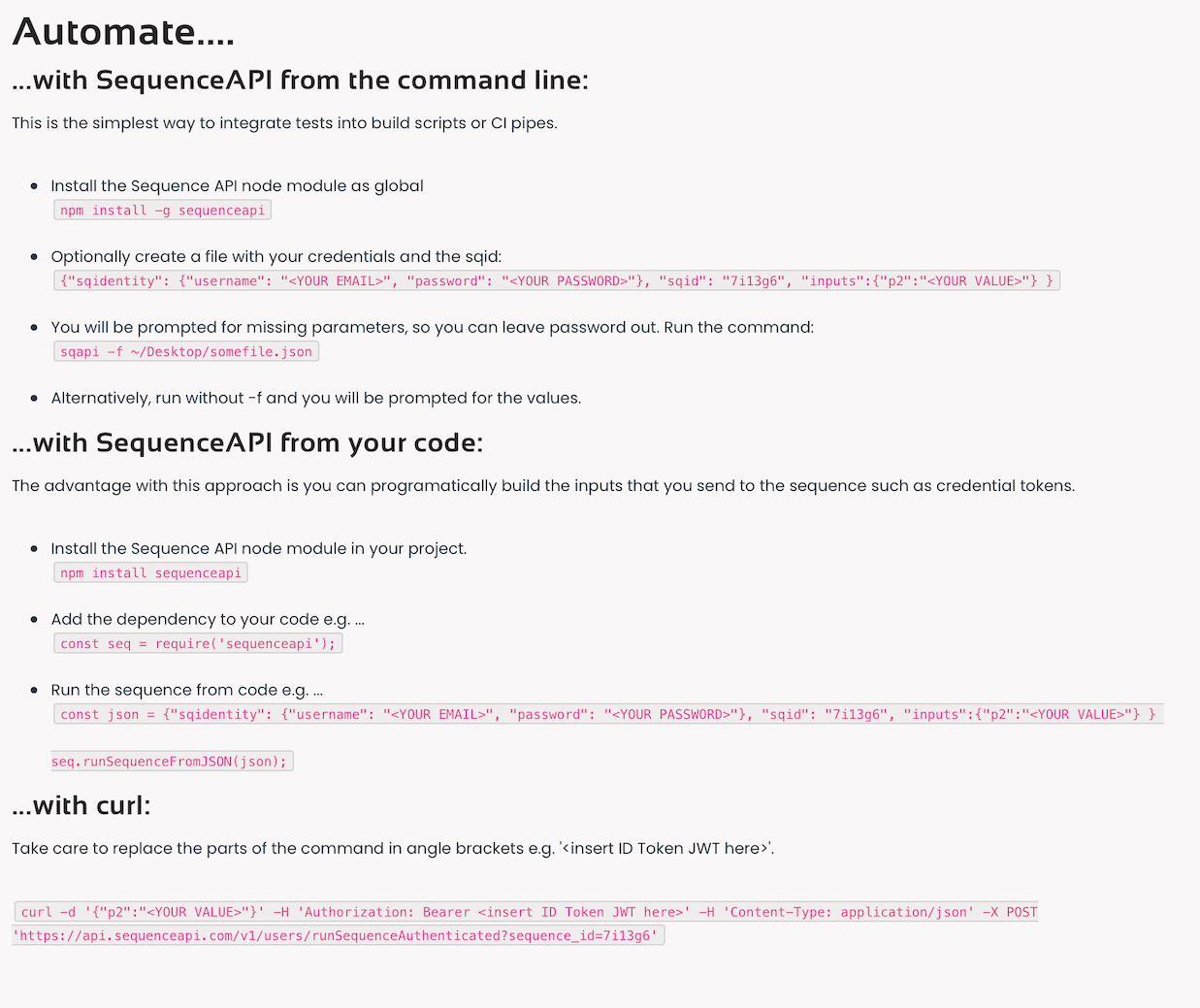
If you click on the automation link from the run, then these commands will be prepopulated with the parameter values of the run:
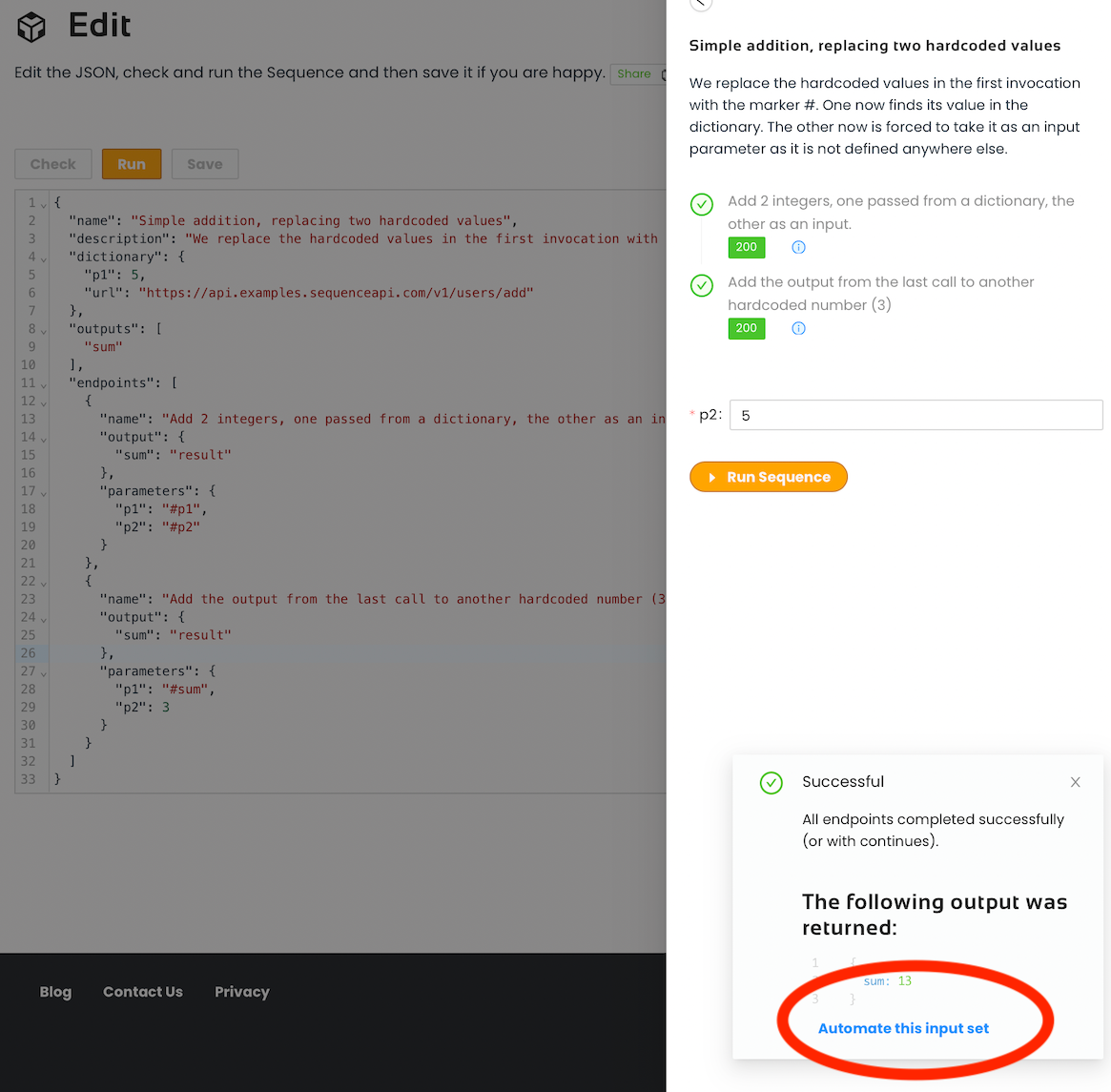
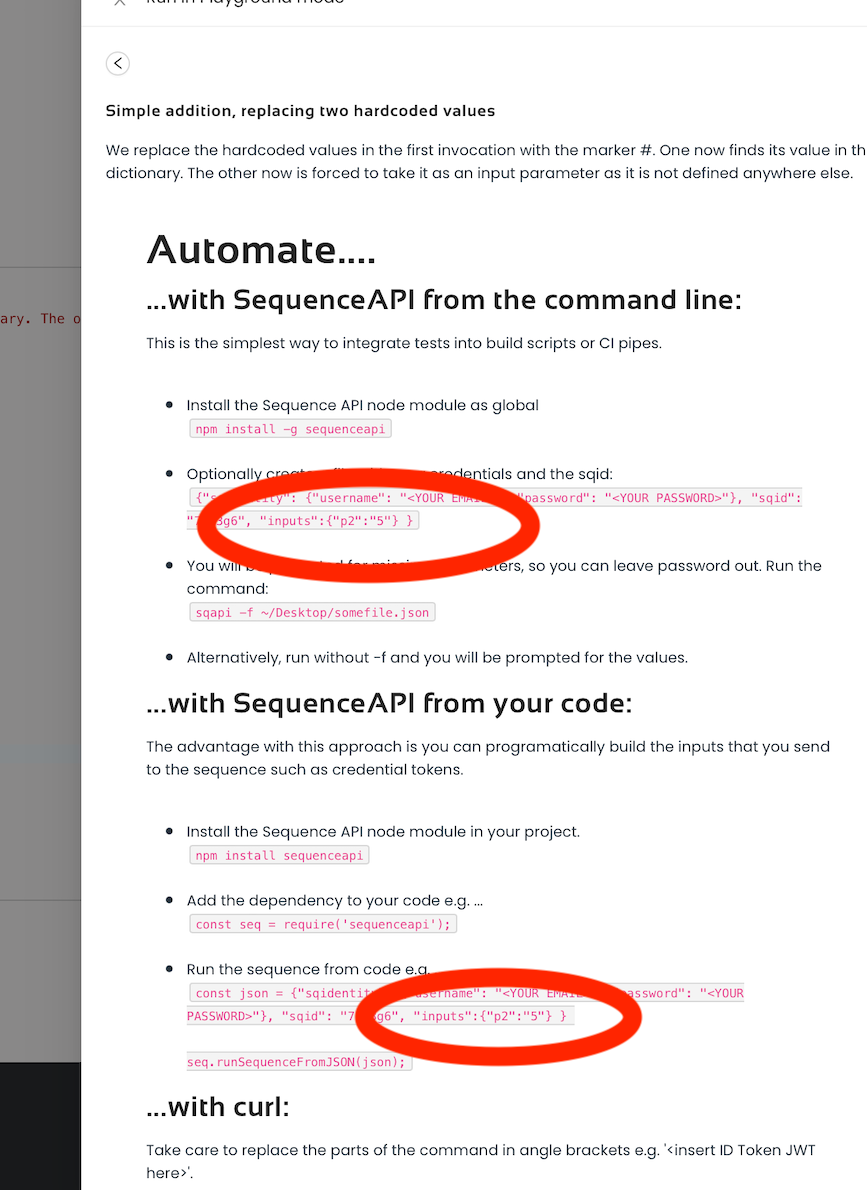
Automating with the command line utility
Install our npm module with a global setting:
npm install -g sequenceapi
You can test this straight away simply by running the command sqapi (or sequenceapi). You will be prompted for your credentials and the ID ofnthe sequence you wish to run (7i13g6):
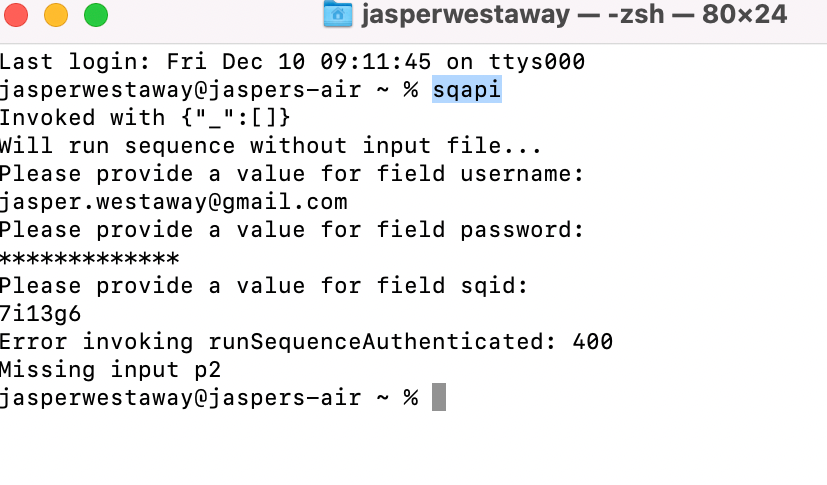
The run failed because we need to provide the input p1. Create a JSON file that at leasts defines p1, but you can add the ID and credentials as well (else it will prompt you):
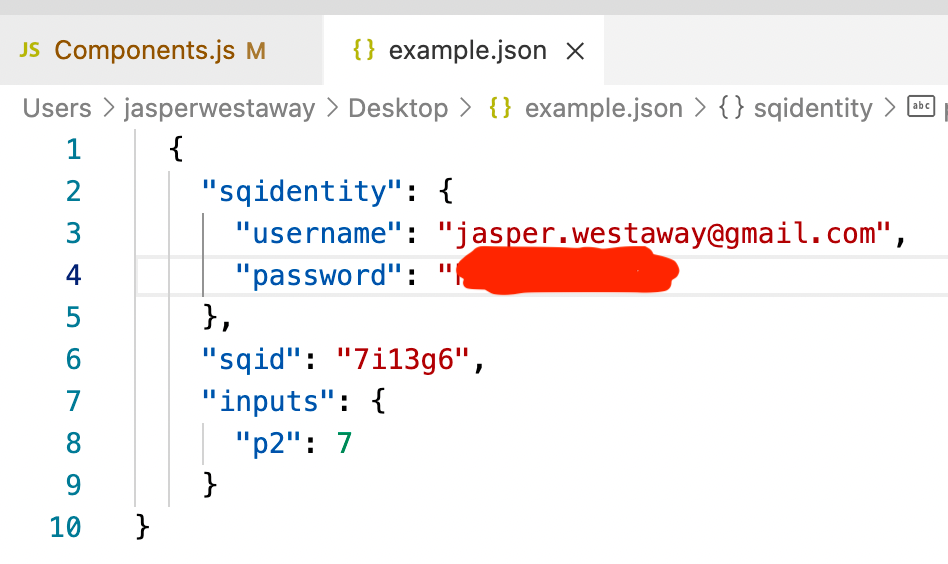
I use the argument -f to pass this file through to the sqapi command:
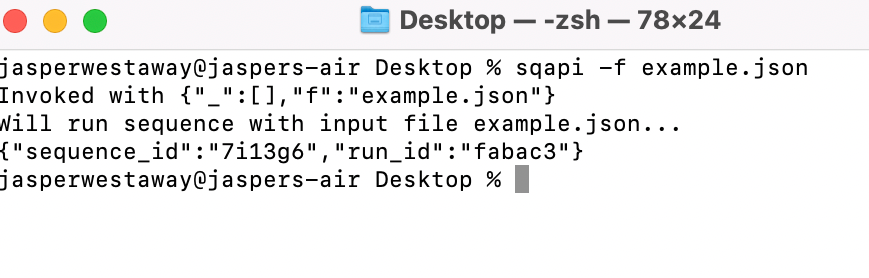
A successful invocatio will return the ID of the sequence and the ID of the run itself. Runs are asynchronous and can take an arbitrary period to complete, so we don't provide the response. Head over to the Runs view in the browser to see the response.
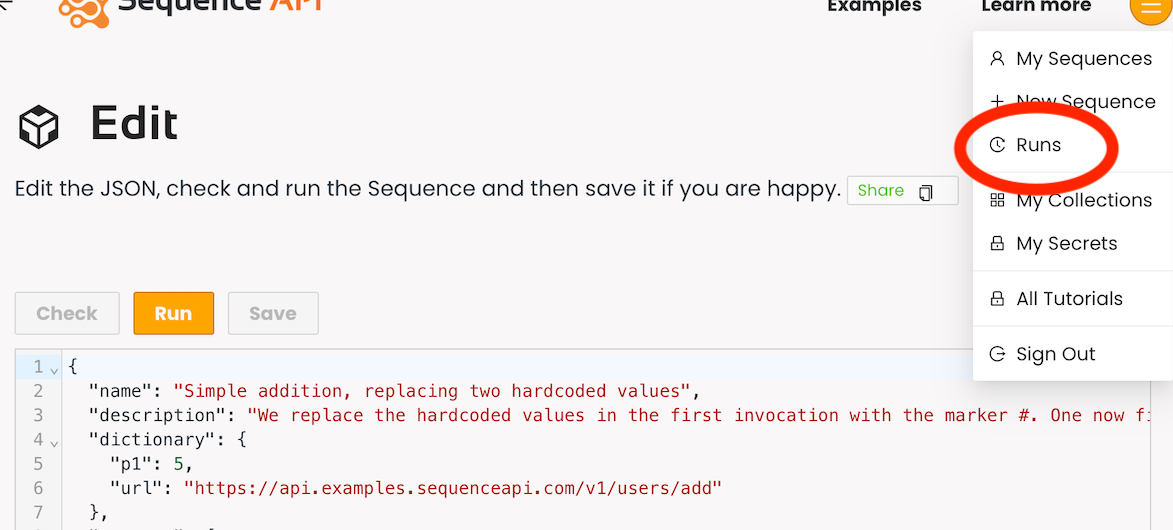
This view is live, so new runs will magically appear. In this case, click on Fetch older runs to recover this run.
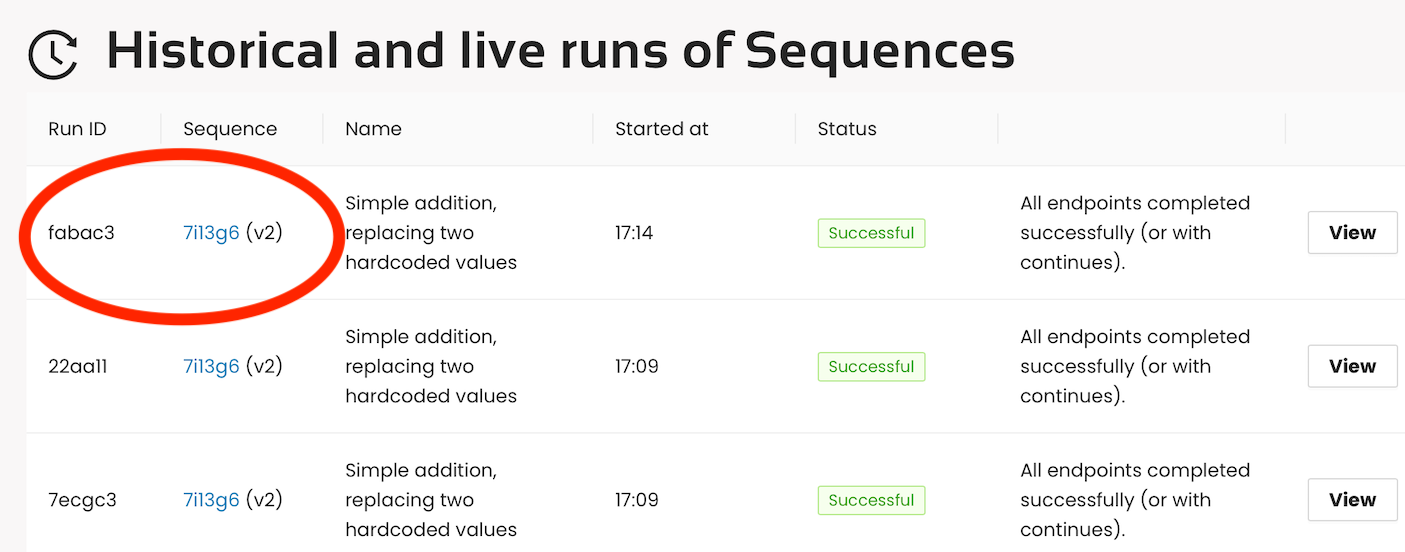
You can click on View to get more details:
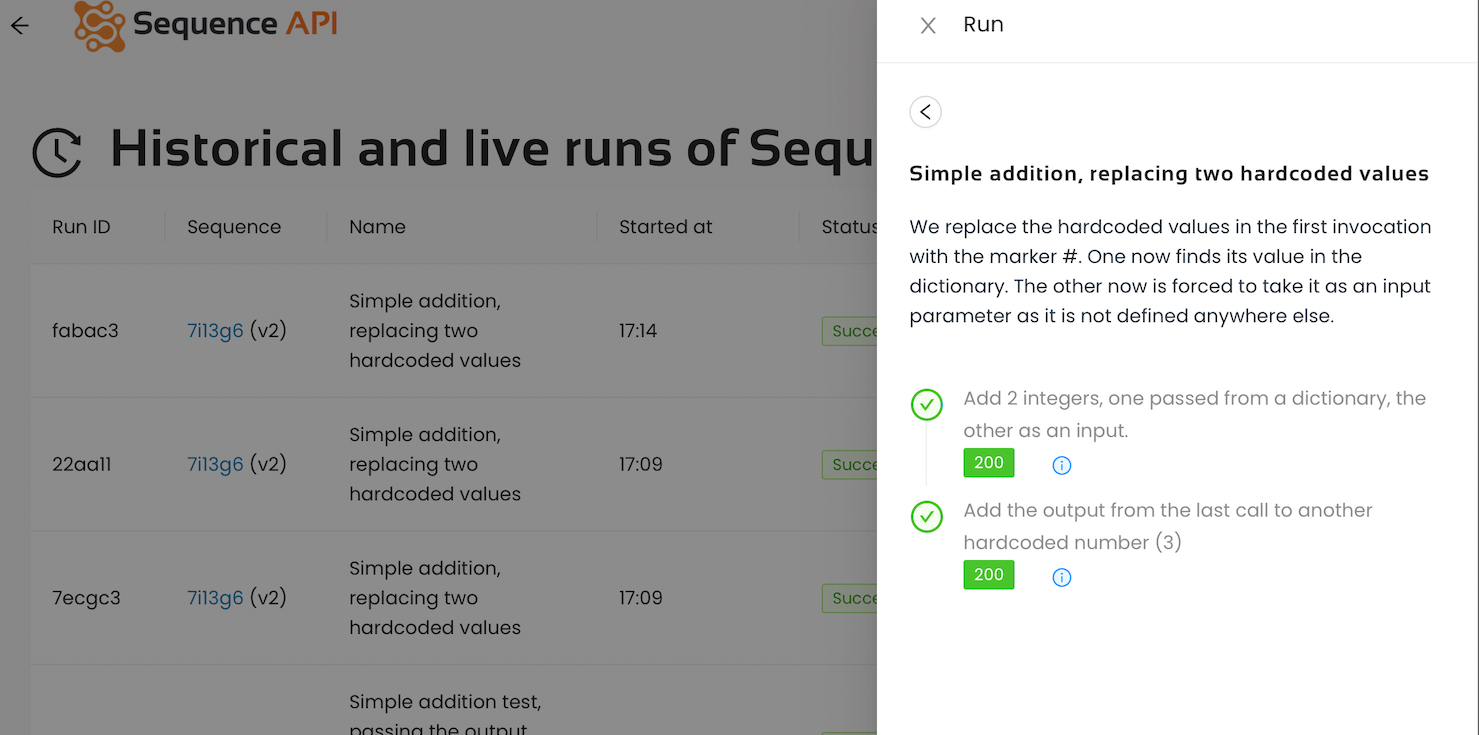
Automating through code integration
In the directory where your code will live, install our node module. The global flag is not required:
npm install sequenceapi
Write your javascript: Below I've highlighted three elements:
- Add the reference to the node module.
- Import any configuration.
- Invoke the run command.
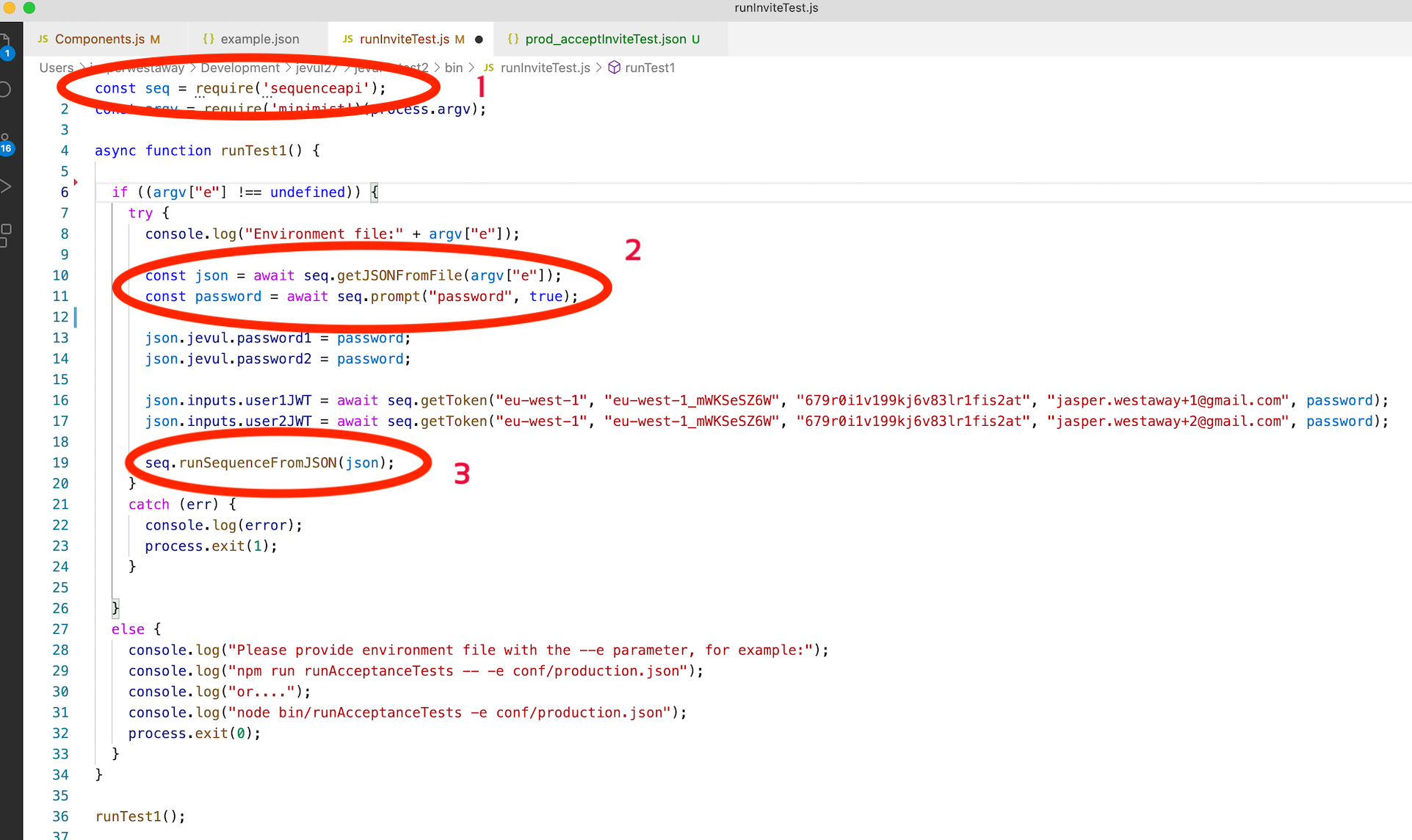
And below is the supporting JSON configuration file:
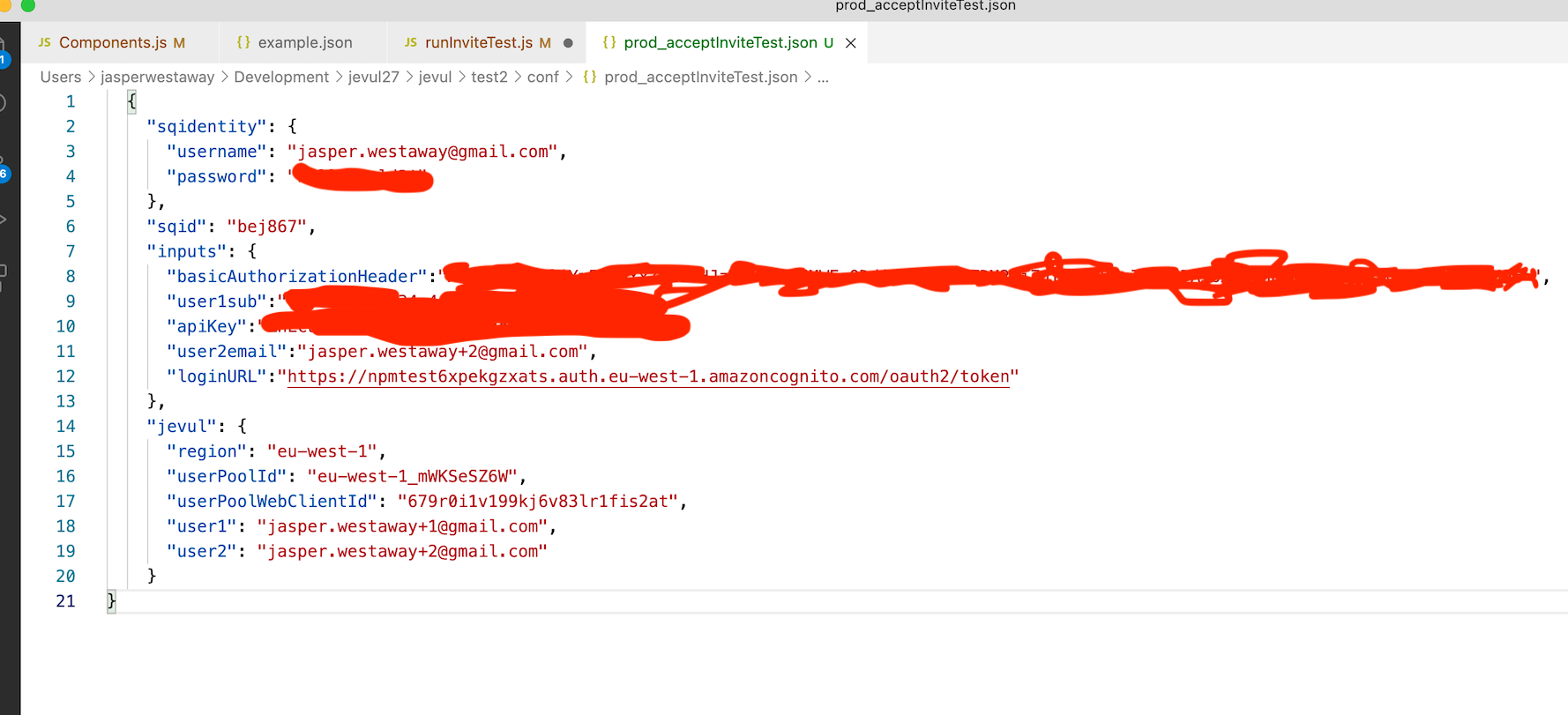
I then run the code through the node command:
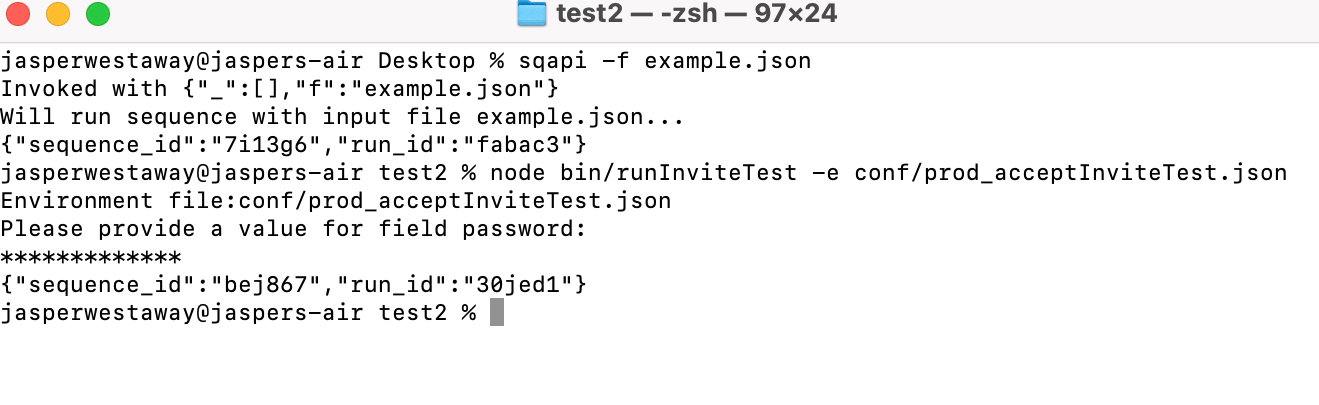
And we can monitor the run in real time:
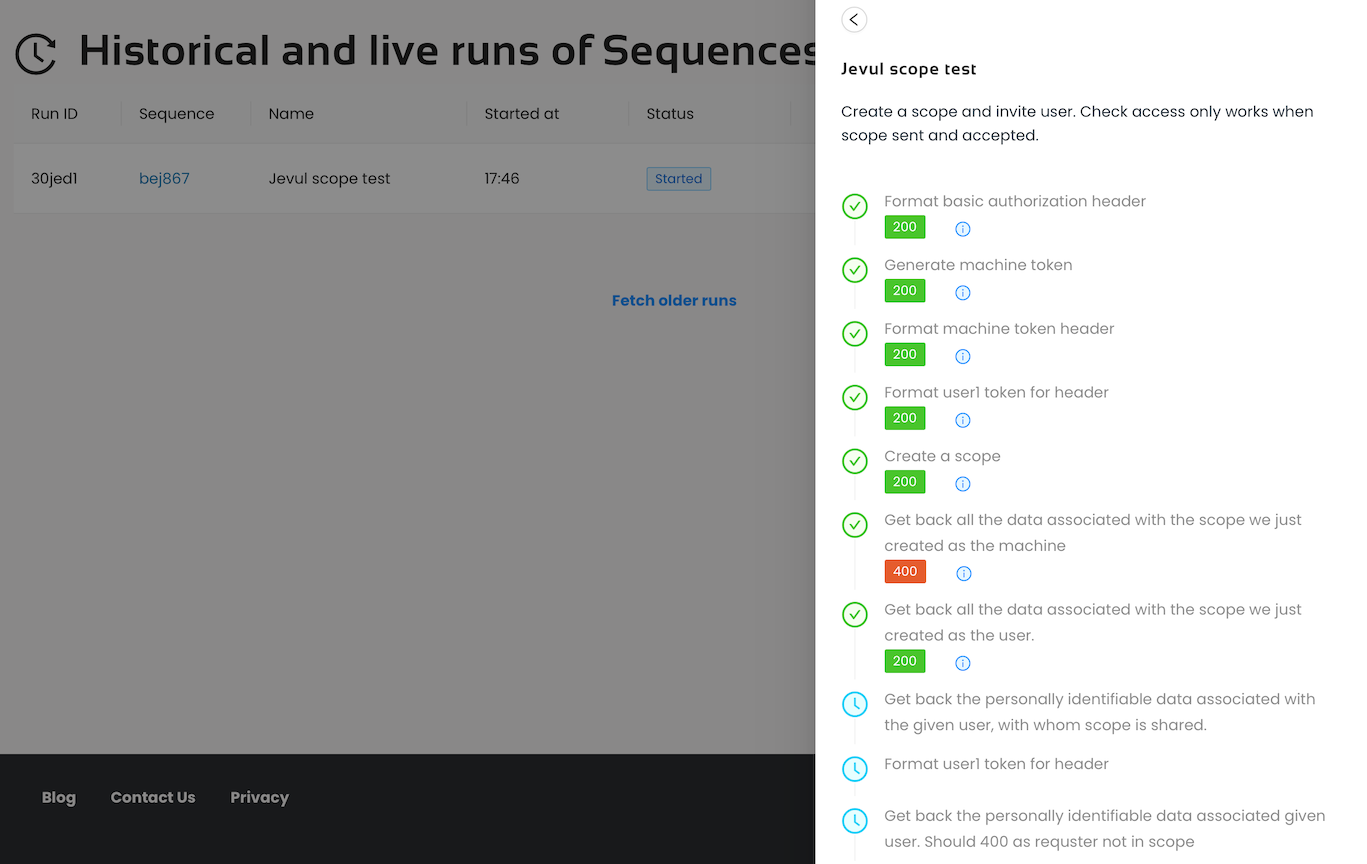
Automating with curl
SequenceAPI is built on AWS lamabdas and API Gateway, so all the commands are available through our own api.
To run our first example with curl then we simply use:
curl -d '{"p2":"5"}' -H 'Authorization: Bearer <insert ID Token JWT here>' -H 'Content-Type: application/json' -X POST 'https://api.sequenceapi.com/v1/users/runSequenceAuthenticated?sequence_id=7i13g6'
Replacing <insert ID Token JWT here>
with the token generated from your username/password. You can generate the token through the command line utility:
sqapi -t <username>
Of course, once the command line utility is installed then you might as well use that, but you can find other ways of generating via AWS such as installing the amplify libraries directly.
Receiving fail notifications
You may not want to check the Runs screen in Sequence API to find out if any automated tests failed. Alternatively you can receive an email notification when a run fails.
To do this you add the parameter notifyonfail=email to the invocation of sequence.
If you are automating from the command line or inside your code then add it to your json configuration file at the top level, e.g.....
{
"sqidentity":{
"username": "jasper.westaway@gmail.com",
},
"sqid": "j7ca50",
"notifyonfail": "email",
"inputs":{
"p2":"1"
}
}
Else, if you are using Curl then add to the url...
curl -d '{"p2":5}' -H 'Authorization: Bearer ' -H 'Content-Type: application/json' -X POST 'https://api.sequenceapi.com/v1/users/runSequenceAuthenticated?sequence_id=7i13g6¬ifyonfail=email'
If this run fails then it will generate an email with an overview of the failure:
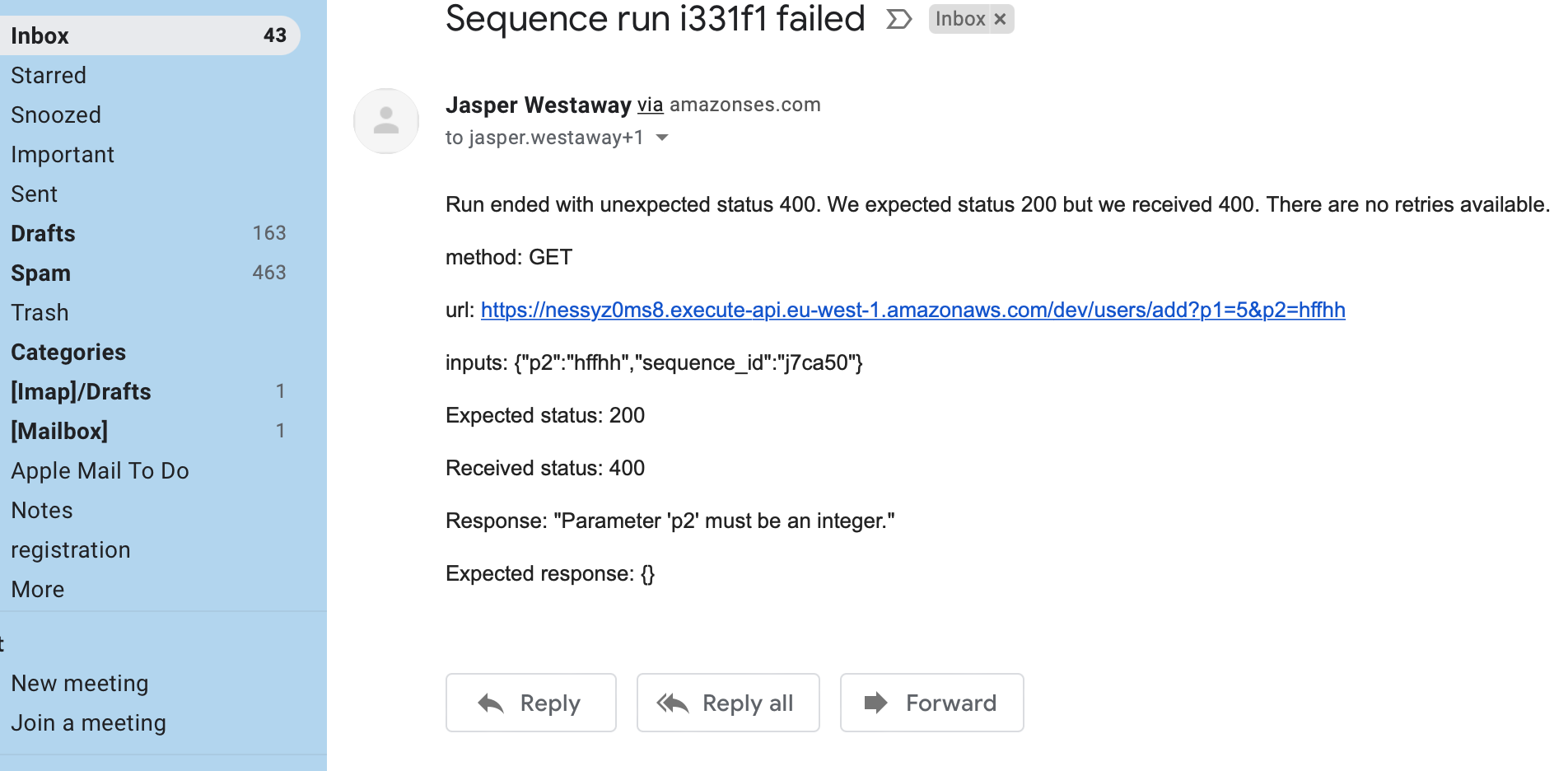